In today’s world of the internet, websites don’t just provide useful information; they also give you a chance to make money. One good way to earn money from your website is by adding Google Ads. In this article, we’ll show you how to make a Psychological Quiz Tool about people’s minds, and we’ll help you put Google Ads into it to make more money.
Table of Contents
Introduction: The Power of Psychological Quiz Tool
Quizzes about how people think and feel are liked by many and make visitors interested and tell us things about their personalities. Before we start talking about Google Ads, let’s create the base of our quiz tool using HTML, CSS, and JavaScript.
Creating the Psychological Quiz Tool HTML Structure (index.html)
<!DOCTYPE html> <html> <head> <title>Psychological Quiz</title> <link rel="stylesheet" href="styles.css"> </head> <body> <div id="quiz-container"> <h2>Psychological Quiz - Human Nature</h2> <br> <div id="question"></div> <br> <div id="options"></div> <script async src="https://pagead2.googlesyndication.com/pagead/js/adsbygoogle.js?client=ca-pub-xxxxxxxxxxxxx" crossorigin="anonymous"></script> <ins class="adsbygoogle" style="display:block; text-align:center;" data-ad-layout="in-article" data-ad-format="fluid" data-ad-client="ca-pub-xxxxxxxxxxxxxxx" data-ad-slot="xxxxxxxxxxxx"></ins> <script> (adsbygoogle = window.adsbygoogle || []).push({}); </script> <button onclick="nextQuestion()">Next</button> </div> <div id="result-container" class="hidden"> <h1>Results</h1> <div id="result"></div> </div> <script src="script.js"></script> </body> </html>
Styling the Quiz (styles.css):
* { margin: 0; padding: 0; box-sizing: border-box; } body { margin: 0; padding: 0; } #quiz-container { max-width: 800px; margin: auto; padding: 20px; background-color: #ffffff; border-radius: 10px; box-shadow: 0 0 10px rgba(0, 0, 0, 0.8); } h2 { text-align: center; color: rgb(0, 0, 0) } #options label { display: block; margin-bottom: 10px } button { display: block; margin: 20px auto 0; padding: 10px 20px; background-color: #007bff; color: #fff; border: none; border-radius: 5px; cursor: pointer; transition: background-color .2s; box-shadow: 0 2px 5px rgba(0, 0, 0, .2) } button:hover { background-color: #0056b3 } #question{ text-align: center; } .hidden { display: none } #result-container { max-width: 800px; margin: auto; padding: 20px; background-color: #ffffff; border-radius: 10px; box-shadow: 0 0 10px rgba(0, 0, 0, 0.8); color: black; }
**Adding Quiz Functionality (script.js):
const quizQuestions = [ { question: "1. How do you typically handle stress?", options: [ "a) I confront the issue directly and try to solve it.", "b) I talk to someone about my feelings.", "c) I tend to keep it to myself and cope on my own.", ], }, { question: "2. When making decisions, I rely more on...", options: [ "a) Logic and reasoning.", "b) Intuition and gut feelings.", "c) Seeking advice from others.", ], }, { question: "3. In social situations, I am...", options: [ "a) Outgoing and enjoy meeting new people.", "b) Reserved and prefer spending time alone.", "c) Somewhere in between, depending on my mood.", ], }, { question: "4. How do you handle criticism?", options: [ "a) I take it constructively and use it to improve.", "b) I feel hurt but try to learn from it.", "c) I become defensive and find it hard to accept.", ], }, { question: "5. What motivates you the most?", options: [ "a) Achieving personal goals and success.", "b) Making a positive impact on others.", "c) Feeling content and at peace.", ], }, ]; let currentQuestion = 0; let score = { a: 0, b: 0, c: 0, }; function displayQuestion() { const questionElement = document.getElementById("question"); const optionsElement = document.getElementById("options"); const currentQuizQuestion = quizQuestions[currentQuestion]; questionElement.textContent = currentQuizQuestion.question; optionsElement.innerHTML = ""; currentQuizQuestion.options.forEach((option) => { const optionElement = document.createElement("label"); optionElement.innerHTML = `<input type="radio" name="option" value="${option.charAt(0).toLowerCase()}">${option}`; optionsElement.appendChild(optionElement); }); } function checkAnswer() { const selectedOption = document.querySelector('input[name="option"]:checked'); if (!selectedOption) { alert("Please select an option."); return; } const selectedAnswer = selectedOption.value; if (selectedAnswer === "a") { score.a++; } else if (selectedAnswer === "b") { score.b++; } else if (selectedAnswer === "c") { score.c++; } currentQuestion++; if (currentQuestion < quizQuestions.length) { displayQuestion(); } else { displayResult(); } } function displayResult() { const quizContainer = document.getElementById("quiz-container"); quizContainer.classList.add("hidden"); const resultContainer = document.getElementById("result-container"); resultContainer.classList.remove("hidden"); const resultElement = document.getElementById("result"); resultElement.innerHTML = ""; const bestOption = getBestOption(); const bestResult = getResultTextForOption(bestOption); const resultText = document.createElement("p"); resultText.textContent = "Based on your responses, your personality leans towards: "; resultElement.appendChild(resultText); const bestResultElement = document.createElement("p"); bestResultElement.textContent = bestResult; resultElement.appendChild(bestResultElement); } function getBestOption() { let bestOption = "a"; if (score.b > score[bestOption]) { bestOption = "b"; } if (score.c > score[bestOption]) { bestOption = "c"; } return bestOption; } function getResultTextForOption(option) { if (option === "a") { return "Option A: You tend to confront issues directly and use logic and reasoning when making decisions. You are goal-oriented and strive for personal success."; } else if (option === "b") { return "Option B: You rely on intuition and gut feelings when making decisions. You are empathetic and care about making a positive impact on others."; } else if (option === "c") { return "Option C: You cope with stress internally and prefer spending time alone. You value inner peace and contentment."; } return "A mix of options."; } function nextQuestion() { checkAnswer(); } // Display the first question on page load displayQuestion();
Conclusion:
In this article, we’ve explored the creation of a psychological quiz tool that engages website visitors while integrating Google Ads for revenue generation. By following these steps, you can offer engaging quizzes to your audience while monetizing your content effectively.
Remember to customize your quiz questions and design to align with your website’s theme and target audience. With a well-executed strategy, you can create an engaging user experience and generate income through Google Ads on your psychological quiz tool. Good luck with your website monetization journey!
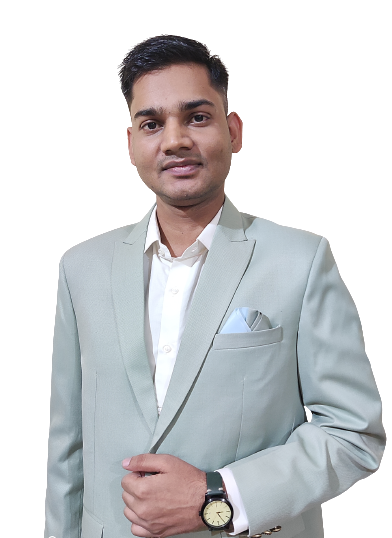
🌟 Unleash the Digital Power with Devendra Gupta 🌟
🖥️ Tech Enthusiast |Software Reviewer| Blogging Expert | SEO Guru |
Web developer 📊
🔍I tested hundreds of Software and reviewed