Hello there, young technologists! Today, we’re going to explore something really cool – creating your very own Image to WebP Converter Tool. Don’t worry; it might sound fancy, but we’ll make it super simple, and you’ll have a fantastic tool to convert images in no time!
Table of Contents
Why WebP?
Firstly, let’s talk about WebP.
On the internet, images look fantastic thanks to this unique image format.It’s like turning your favorite photos into internet superheroes with superhuman speed. S
What You’ll Need
To make this magic happen, we’ll need HTML, CSS, and JavaScript.. Think of them as the building blocks of our tool. HTML makes the structure, CSS makes it look awesome, and JavaScript adds the smarts.
Step 1: Building the Base (HTML)
Imagine the HTML part as the foundation of a house. Here’s a simple start:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Image to WebP Converter</title> <link rel="stylesheet" href="styles.css"> </head> <body> <div class="container"> <h1>Image to WebP Converter</h1> <input type="file" id="imageInput" accept="image/*"> <button id="convertButton" class="green-button">Convert to WebP</button> <div id="output" class="image-preview"></div> </div> <script src="script.js"></script> </body> </html>
Step 2: Making It Look Awesome (CSS)
After that, let’s style it with CSS. It’s like giving our tool a fancy coat. Here’s a start; you can make it even cooler:
body { margin: 0; font-family: Arial, sans-serif; background-color: #f0f0f0; } .container { max-width: 600px; margin: 0 auto; padding: 20px; background-color: white; border-radius: 10px; box-shadow: 0px 0px 10px rgba(0, 0, 0, 0.1); } h1 { text-align: center; } input[type="file"] { display: block; width: 100%; margin: 20px 0; } .green-button { background-color: #00cc66; color: white; border: none; padding: 10px 20px; border-radius: 5px; cursor: pointer; } .image-preview { text-align: center; margin-top: 20px; } .image-preview img { max-width: 100%; border: 1px solid #ccc; border-radius: 5px; box-shadow: 0px 0px 5px rgba(0, 0, 0, 0.1); display: block; margin: 0 auto; }
Step 3: Adding the Brains (JavaScript)
Now, it’s time to add the smarts with JavaScript. This part listens to what you tell it to do. We’ll use it to convert images to WebP format. Here’s how it could begin:
const imageInput = document.getElementById('imageInput'); const convertButton = document.getElementById('convertButton'); const output = document.getElementById('output'); convertButton.addEventListener('click', async () => { if (!imageInput.files.length) { return; } const imageFile = imageInput.files[0]; const image = new Image(); image.src = URL.createObjectURL(imageFile); image.onload = async () => { const canvas = document.createElement('canvas'); canvas.width = image.width; canvas.height = image.height; const ctx = canvas.getContext('2d'); ctx.drawImage(image, 0, 0); const compressedWebP = await convertToWebP(canvas, 0.1); const webPImage = new Image(); webPImage.src = compressedWebP; output.innerHTML = ''; output.appendChild(webPImage); const downloadButton = document.createElement('a'); downloadButton.textContent = 'Download WebP'; downloadButton.href = compressedWebP; downloadButton.download = 'converted_image.webp'; output.appendChild(downloadButton); }; }); async function convertToWebP(canvas, quality) { return new Promise((resolve) => { canvas.toBlob( (blob) => { resolve(URL.createObjectURL(blob)); }, 'image/webp', quality ); }); }
Bringing It All Together
Now, when you open your HTML file in a web browser, you’ll see your tool’s basic structure. You can expand the features and enhance the aesthetic by tinkering with the JavaScript and CSS elements.
Watch Video for More
It’s Your Turn!
It’s your chance now to be a technological wizard. Experiment with HTML, CSS, and JavaScript to add interest to your tool.You may find out more about these languages online, ask questions, and look into other interesting projects.
By creating your Image to WebP Converter Tool, you’re taking a step into the world of web development. Remember, even the greatest tech wizards started with simple projects. Have fun coding, and who knows, you might invent the next big thing on the internet!
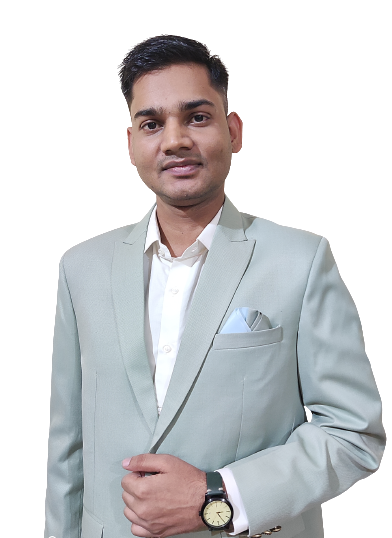
🌟 Unleash the Digital Power with Devendra Gupta 🌟
🖥️ Tech Enthusiast |Software Reviewer| Blogging Expert | SEO Guru |
Web developer 📊
🔍I tested hundreds of Software and reviewed